Mesibo Open Source Chat, Voice and Video Calls UI Modules
mesibo provides a variety of pre-built open-source UI components that allow you to quickly add messaging, voice & video calls, and conferencing graphical user interface in your app in just a few lines of code. mesibo UI components are optional and provided to aid in the app development process. You can completely design your own UI components using mesibo core APIs.
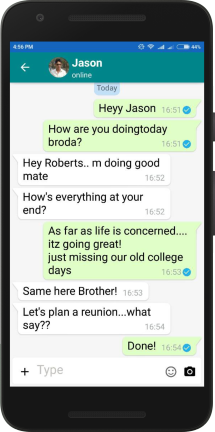
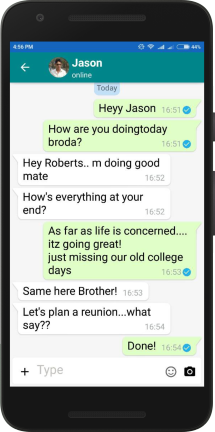
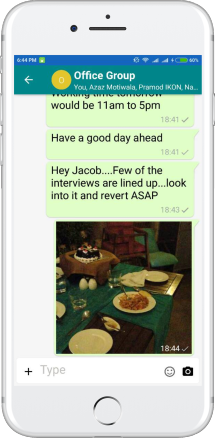
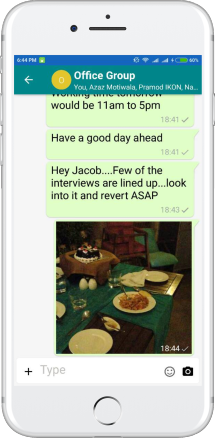
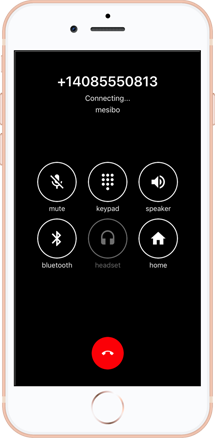
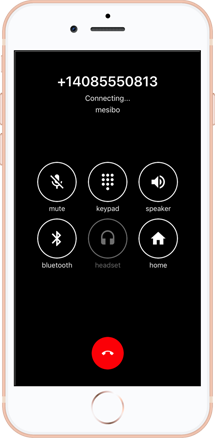
mesibo UI modules are completely customizable. You can not only customize colors, icons, etc but also customize how each message is rendered. This enables you to create powerful apps, and chatbots in no time. In addition to that, the entire source code of UI modules is available on GitHub if you need to customize them as per your needs
Since each App has different UI requirements, we DO NOT claim that these UI modules will meet all your needs. However, they can get you started quickly and since they are open-source, you can download the entire source code of UI modules and modify it to suit your needs.
Using UI Modules
In this document, we will briefly describe how to use and customize UI modules.
Note that, mesibo UI modules are developed by our partners and hence, unlike mesibo APIs,
- They may not follow the common API signature and other mesibo coding guidelines
- You will find some differences in Android and iOS UI modules. Refer to the source code.
Hence, use this document as a high-level reference only. The best way to learn how to use mesibo UI modules is to refer to the open-source messenger app for Android, iOS, and Javascript source code which uses mesibo UI modules for various functionalities like welcome screen, login, media picker, one-to-one and group messaging, user list, group management, showing profiles, etc.
Type of UI Modules
Following are types of UI modules, you can use one or more as required by you:
- Messaging - UI module for messaging similar to WhatsApp/Telegram
- Call and Conferencing - Video and Voice Calls, and Conferencing
- MediaPicker - UI module to select images, video, files, etc. from the phone, and optionally to crop, and rotate. Messaging module uses the MediaPicker module.
- Helper - UI module for login and welcome screens
UI Modules Source Code
You can download the entire source code from GitHub. Refer to the source code page to know about all mesibo source code repositories.
Android UI Modules
iOS UI Modules
Messaging UI
There are primarily two types of Messaging UI screens (fragment in Android, UIViewController in iOS):
- Messaging Screen, which renders all the messages for a particular user or a group and allows users to send messages, images, videos, files, etc.
- User List Screen, which displays a list of users in different modes. The modes are explained below.
The different modes for User List
are:
MODE_MESSAGES
shows a list of the latest messages, one per user, sorted by time. This is generally a start screen for messaging.MODE_CONTACTS
shows contacts - a list of Mesibo Users in the applicationMODE_FORWARD
shows a list of users to forward the message(s)MODE_GROUPS
shows a list of users to form a groupMODE_EDITGROUP
shows UI for editing different attributes of a group such as - Group Member List, Group Profile Picture, Group Title, or Group Name
Please refer to the first app source code available on GitHub - First Mesibo App for Androidopen_in_new and First Mesibo App for iOSopen_in_new to learn how to use UI modules.
Launching Messaging UI in Android
Install Mesibo UI Modules by following Instructions for Android
Import the messaging-UI Module.
import com.mesibo.messaging.MesiboUI;
Once you have imported, you can either create the following fragments and use them in your app or use utility functions to launch UI.
MesiboUserListFragment
, User List Fragment as mentioned above.MesiboMessagingFragment
, Messaging Fragment as mentioned above.
Alternatively, you can launch UI by calling:
MesiboUserListScreenOptions launchOptions = new MesiboUserListScreenOptions();
MesiboUI.launchUserList(this, launchOptions);
You can also launch chat UI for a particular user or a group directly. For example,
In Java and Kotlin,
MesiboMessageScreenOptions launchOptions = new MesiboMessageScreenOptions();
launchOptions.profile = profile.
MesiboUI.launchMessaging(this, launchOptions);
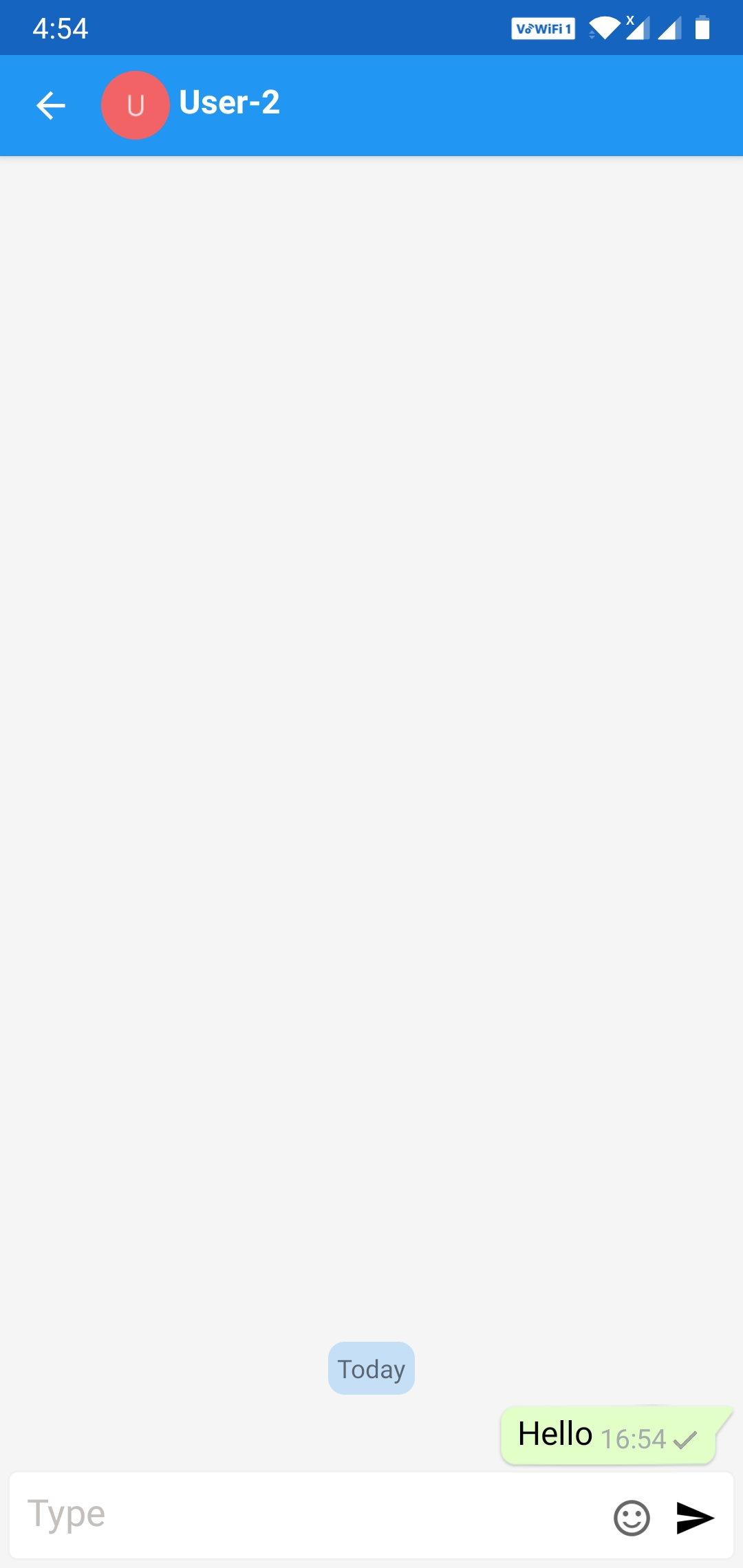
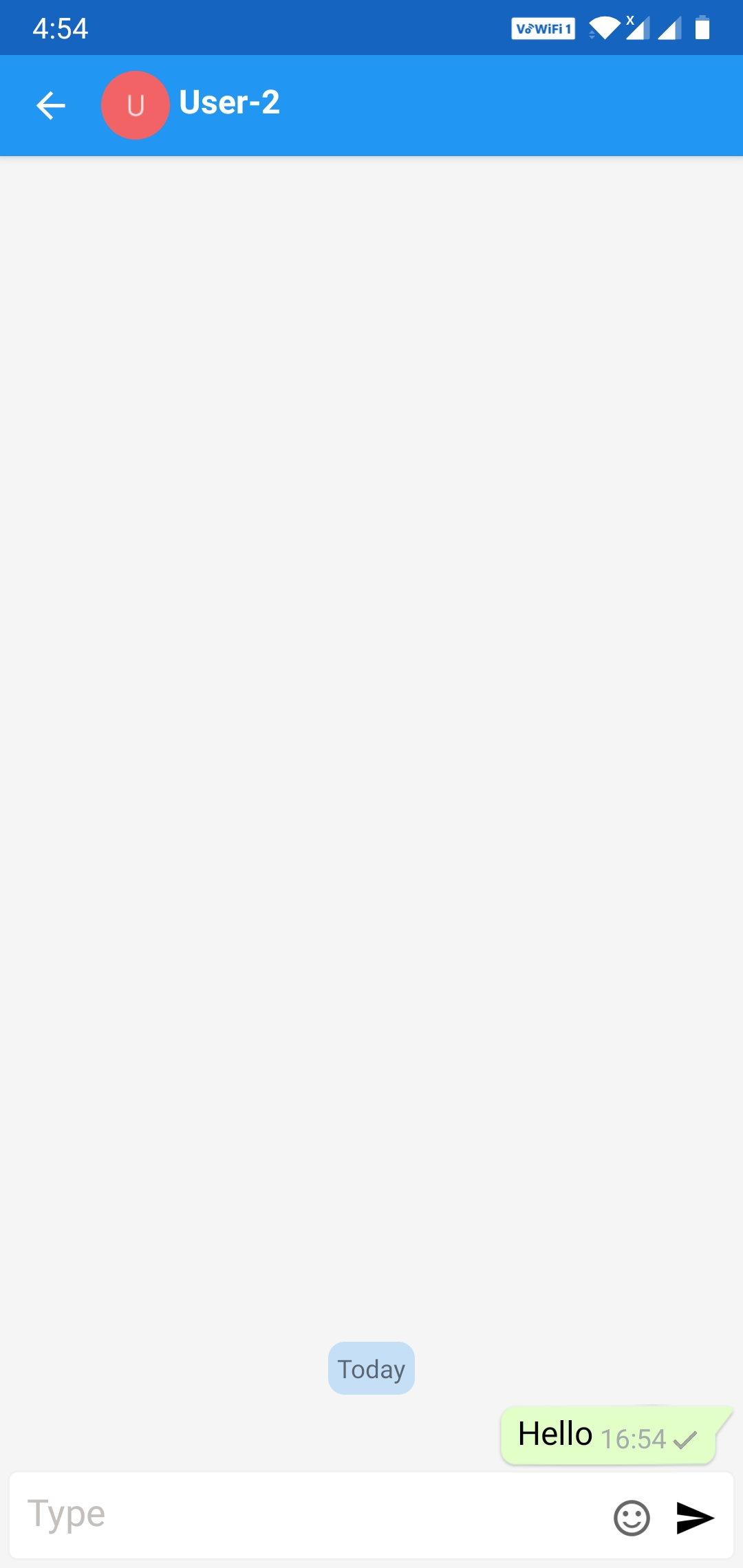
Launching Messaging UI in iOS
Install Mesibo UI Modules by following Instructions for iOS
Import the Mesibo UI Module.
In Objective-C,
#import "MesiboUi/MesiboUi.h"
In Swift,
import MesiboUI
You can then get User List Controller by
let mesiboController = MesiboUI.getUserListViewController()
You can also launch chat UI for a particular user or a group directly. For example,
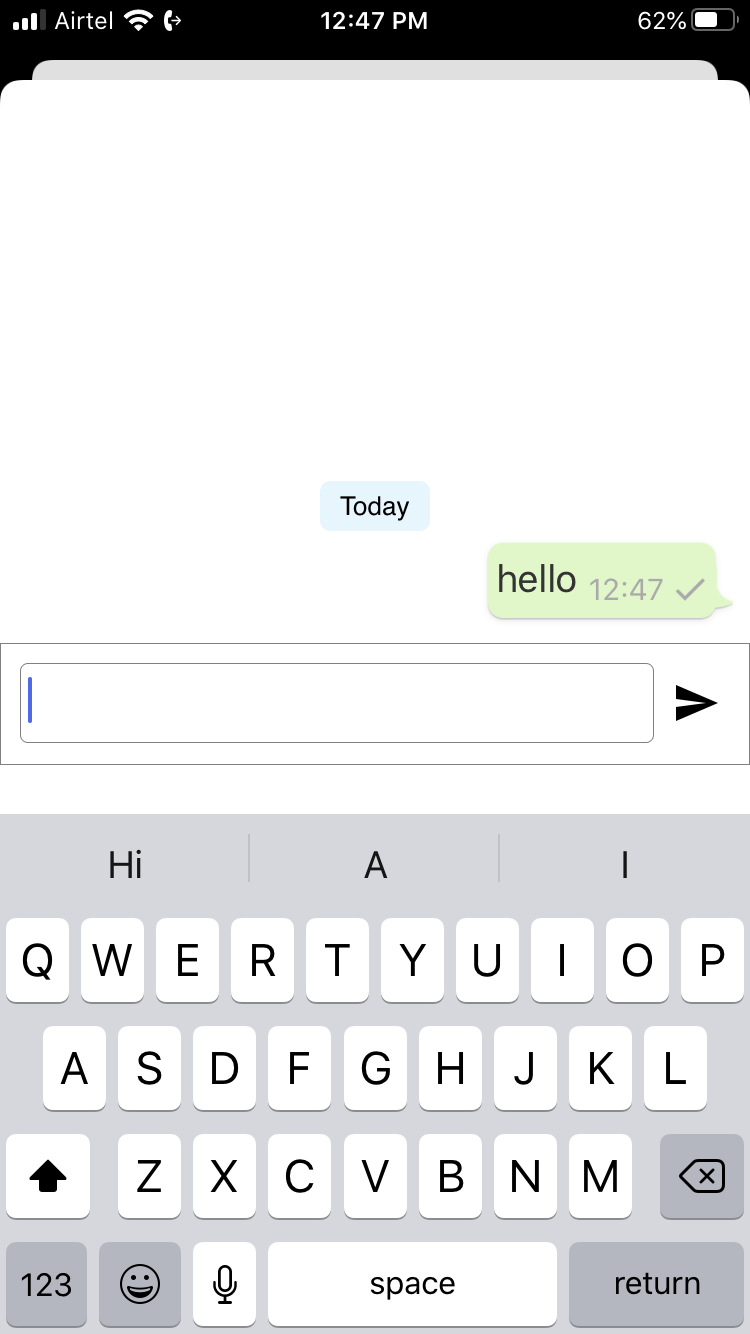
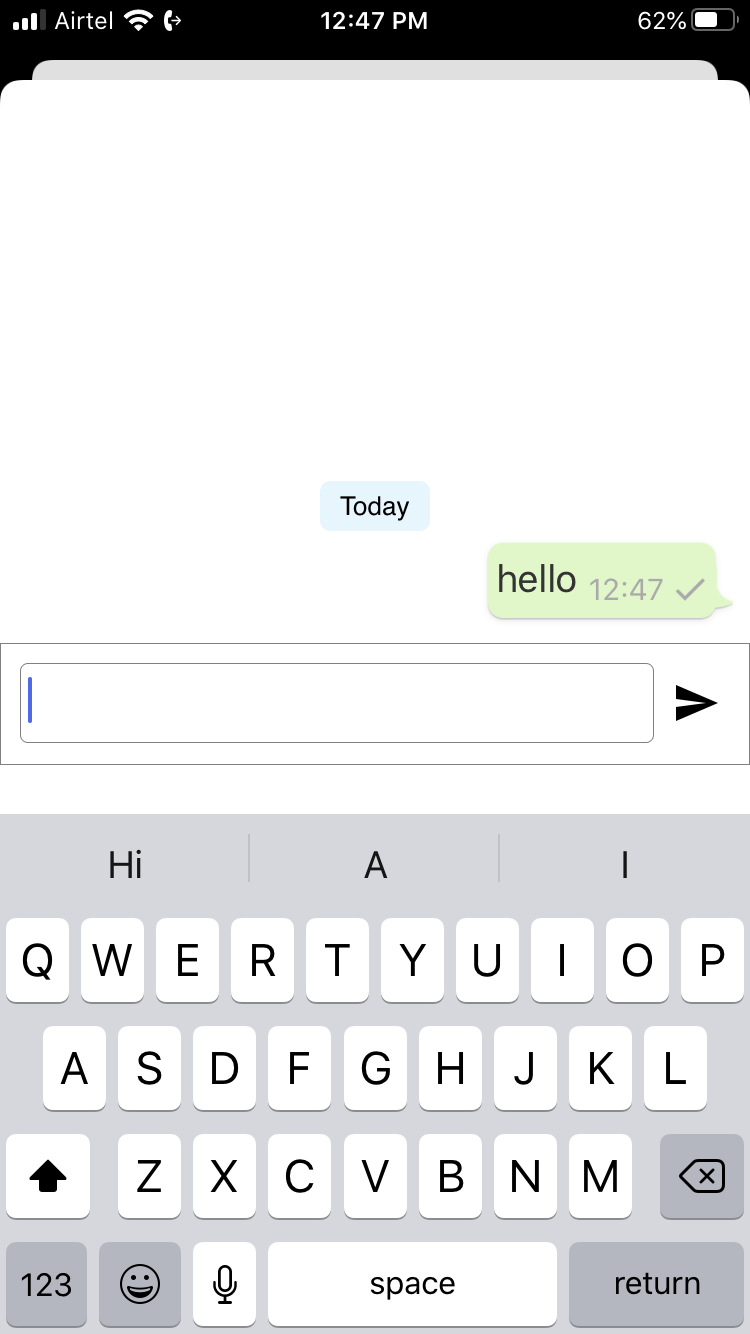
To launch the messaging UI for a user, you need to pass the address for that user.
MesiboMessageScreenOptions *opts = [MesiboMessageScreenOptions new];
opts.profile = profile;
opts.navigation = YES;
[MesiboUI launchMessaging:self opts:opts];
var opts:MesiboMessageScreenOptions = MesiboMessageScreenOptions();
opts.profile = profile;
opts.navigation = true;
MesiboUI.launchMessaging(self, opts:opts)
In the next section, we will learn how to custom buttons to UI screens and customize the way messages are rendered.