Publishing a Profile and Controlling Profile Privacy
Your app users can create and publish a profile about themself so other users can view it. Depending on the app, users can add different information to the profile like name, photos, location, relationship status, etc. In addition, group owners and admins can also create, edit and publish profiles for their groups with information about the group. Note that a user can't modify or publish another user's profile.
In this section, we'll explore:
- How a user can set profile fields and publish them so other users can access their profile.
- How a user can control the privacy of their profile at different levels so different groups of people can access different information.
Publishing a Profile
To publish a user profile, you need to get MesiboSelfProfile
object and set the required information in it.
MesiboSelfProfile selfProfile = mesibo.getSelfProfile();
Similarly, to publish a group profile, the group owner or admin needs to get the profile object for that group.
MesiboProfile profile = mesibo.getProfile(groupId);
After getting the profile object, you can call the following functions to set any information suitable for your app.
Setting Profile Information
mesibo stores all profile information as name-value pairs. You can use any number of name-value pairs (up to a total size of 16KB) to store profile data.
void profile.setString(name, value); // sets a string value
void profile.setInt(name, value); // sets an integer number value
void profile.setLong(name, value); // sets a long number value
void profile.setDouble(name, value); // sets a real number value
void profile.setBoolean(name, value); // sets a true/false value
For example,
MesiboSelfProfile selfProfile = mesibo.getSelfProfile();
selfProfile.reset(); // clear existing information
selfProfile.setString("status", "Hey! I am using this app.");
selfProfile.setString("city", "San Francisco");
selfProfile.setInt("zip", 94105);
selfProfile.save(); // publish
Every time you save a profile, it will publish the updates and notify all subscribers.
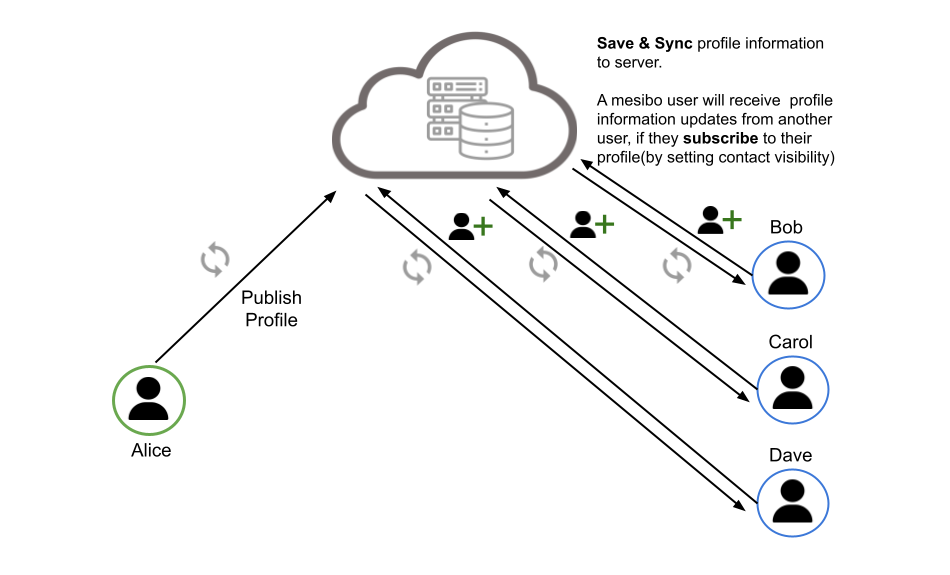
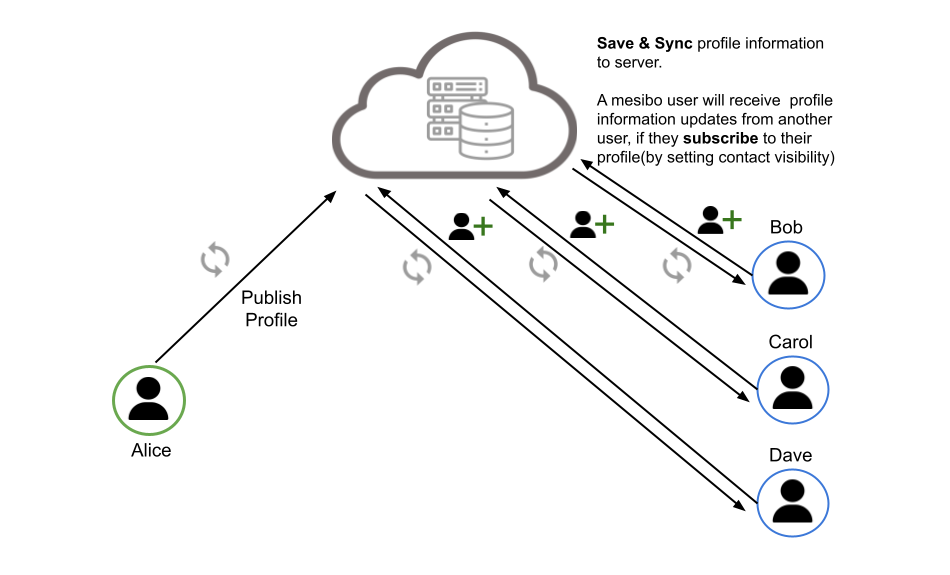
Setting Profile Name
mesibo has dedicated functions to set a name since it's commonly used. You can set various name types:
void profile.setName(user_name);
mesibo also allows you to temporarily override the name from an alternate source like phone contacts. You can also set a temporary name to use if the regular name or overridden is empty.
void profile.setTemporaryName(user_name);
void profile.setOverrideName(user_name);
You can use these functions on any profile, not just the self profile or the group profile.
Setting Profile Images
mesibo allows you to add multiple images to a profile. You can add images using an index number to later retrieve that image.
There are three ways you can add images:
By providing actual image object (Bitmap in Android, UIImage in iOS):
void profile.setImage(image, index);
By providing a valid image URL:
void profile.setImageUrl(url, index);
or, by providing the valid file path of the image
void profile.setImageFromFile(path, index);
When setting a profile image using setImage()
or setImagePath()
, mesibo will automatically upload the image to a file server and generate a URL for it. If you have set a custom
file transfer handler, mesibo will use that. Otherwise, it will use the default file handler to upload the images.
Once the upload completes, mesibo will save the generated image URL in the profile object before publishing it.
When another user accesses your profile, mesibo will download the image from the URL and cache it locally. It also automatically generates a thumbnail image which can be retrieved using getThumbnail().
mesibo resizes the full images when uploading. It also sends a thumbnail for the image at index 0. You can customize the image and thumbnail resize settings using MesiboProfile.setImageProperties()
and MesiboProfile.setThumbnailProperties()
.
Controlling Profile Access Level and Privacy
By default, any profile fields you set are public. However, you can control the privacy of each field so only certain people can access them.
For example, on a social app like Facebook, you can set privacy so only friends see certain photos, while the public sees limited info. In a work app, colleagues might see basic info while managers see more details and HR could see other specifics.
You can do this by adding an access level to each field, like:
profile.setString("city", "Palo Alto", 0); // access level 0, public
profile.setInt("birthYear", 1990, 1); //access level 1
profile.setBoolean("xyz", true, 2); //access level 2
profile.setImage(image, index, 1); // access level 1
0
is public access level. So in above example, "city" is public, while "birthYear" is accessible only to users with access level 1
, while "xyz" is accessible only to users with access level 2
.
You can then assign access levels to a user, for example:
anotherUserProfile.setProfileAccessLevels([1,2,5]);
We will later describe how to set access levels for multiple contacts in the profile synchronization section. Note that, all users whom you have paired have at least access level 1 assigned to them.
You can define up to 8 levels of access controls (or 15 levels on on-premise) which allows you to fine control every profile information to only a designated set of people.
Profile Publishing Mode
By default, when a user publishes their profile, it synchronizes immediately and all subscribers are notified right away. However, the user can change this to on-demand
publishing by setting the publishing mode:
Real-Time Publishing (default)
When the user publishes their profile, the updates are pushed out in real-time and all subscribed users are notified immediately.
Lazy / On-demand Publishing
When the user publishes, their profile is updated on the server but other users are not notified immediately. Instead, updates are pulled by users only when they view the profile. On-demand publishing uses less bandwidth since profile changes are not pushed to all subscribers instantly. Updates are only pulled when users access the profile.
profile.setSyncType(MesiboProfile.SYNCTYPE_REALTIME);
Resetting a Profile
The user has two options for updating the profile.
One option is to remove all existing information by resetting the profile before publishing. The other option is to publish without resetting the profile. In this case, any new name-value pairs will either overwrite existing pairs with the same name, or add new pairs if that name doesn't exist yet.
To reset the profile, the user can call:
profile.reset()
This will clear all existing information before publishing, so the published profile contains only the newly set information.
Saving a Profile
You also save a profile locally to the local database using the save
method.
profile.save()
There are three possibilities when you are calling save
on a profile object:
- If the profile is a self profile, the
save
method saves the profile to the local database and then publishes the latest profile to the server. - If the profile is a group profile and you have modification permission, the
save
method saves the profile to the local database and then publishes the latest profile to the server. - Otherwise, the
save
method saves the profile into the local database.